Mastering RxJS fromEvent: Building Event-Driven Reactive Applications
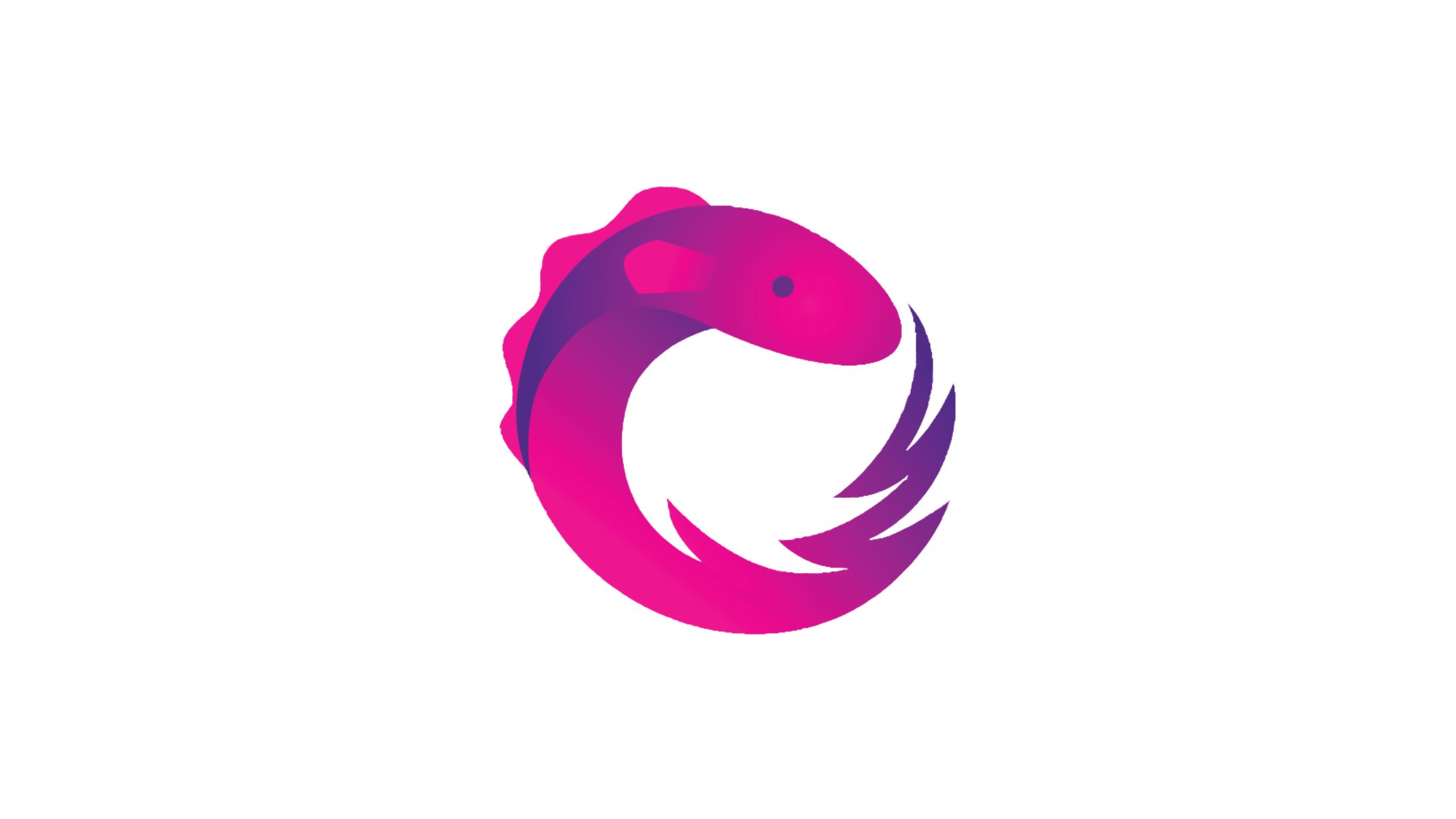
RxJS is a powerful library for reactive programming in JavaScript, and one of its key operators is fromEvent
. The fromEvent
operator allows developers to create Observables from different types of events, such as DOM events, Node.js event emitters, or even custom events. In this guide, we will explore the fromEvent
operator in RxJS, its syntax, and a wide range of use cases. By understanding how to effectively leverage fromEvent
, you will be able to easily build event-driven applications, creating dynamic and interactive experiences for your users.
Understanding RxJS fromEvent
The fromEvent
operator is a fundamental part of RxJS. It transforms events into Observables, enabling you to work with event streams reactively. We will cover the syntax of fromEvent
and explore its parameters, including the event source and an optional event selector. We will also discuss the concept of hot and cold Observables and how it applies to fromEvent
.
import { fromEvent } from 'rxjs';
const button = document.querySelector('#myButton');
const buttonClick$ = fromEvent(button, 'click');
buttonClick$.subscribe(() => {
console.log('Button clicked!');
});
Creating Observables with fromEvent
To use fromEvent
, we need to create an Observable from specific event sources. We will cover various event sources, such as DOM elements, Node.js event emitters, and custom event emitters. We will demonstrate how to create Observables using fromEvent
for each of these sources and how to handle the emitted values.
import { fromEvent } from 'rxjs';
// Creating an Observable from a click event on a DOM button
const button = document.querySelector('#myButton');
const buttonClick$ = fromEvent(button, 'click');
buttonClick$.subscribe(() => {
console.log('Button clicked!');
});
// Creating an Observable from a keyboard event
const input = document.querySelector('#myInput');
const inputKeyup$ = fromEvent(input, 'keyup');
inputKeyup$.subscribe((event) => {
console.log('Key pressed:', event.key);
});
// Creating an Observable from a custom event
const customEmitter = new EventEmitter();
const customEvent$ = fromEvent(customEmitter, 'customEvent');
customEvent$.subscribe(() => {
console.log('Custom event emitted!');
});
customEmitter.emit('customEvent');
Advanced Techniques with fromEvent
fromEvent
offers a rich set of possibilities for manipulating event streams. We will explore advanced techniques such as combining multiple events using operators like merge
, concat
, or race
. We will also discuss the use of operators like debounceTime
, throttleTime
, and distinctUntilChanged
to control the rate of emitted events.
import { fromEvent } from 'rxjs';
import { debounceTime, distinctUntilChanged } from 'rxjs/operators';
const input = document.querySelector('#myInput');
const inputKeyup$ = fromEvent(input, 'keyup');
inputKeyup$
.pipe(
debounceTime(300),
distinctUntilChanged()
)
.subscribe((event) => {
console.log('Typed text:', event.target.value);
});
Error Handling and Resource Cleanup
Error handling is an important aspect of event-driven programming. We will explore how to handle errors that may occur during event processing, including strategies like using the catchError
operator. Additionally, we will cover proper resource cleanup techniques to avoid memory leaks and ensure the cleanup
of event subscriptions using unsubscribe
or other release strategies.
import { fromEvent } from 'rxjs';
import { catchError } from 'rxjs/operators';
const button = document.querySelector('#myButton');
const buttonClick$ = fromEvent(button, 'click');
buttonClick$
.pipe(
catchError((error) => {
console.error('Error:', error);
// Perform error handling logic
return throwError('An error occurred.');
})
)
.subscribe(() => {
console.log('Button clicked!');
});
Real-World Use Cases
In this section, we will dive into real-world use cases where fromEvent
shines. We will cover scenarios such as handling user interactions, implementing autocomplete functionalities, creating drag and drop interfaces, and integrating with third-party libraries that emit events. By exploring these practical examples, you will gain a deeper understanding of how to apply fromEvent
in your own projects.
Conclusion
The RxJS fromEvent
operator is a versatile tool that empowers developers to work with event streams reactively. By understanding its syntax, creating Observables from different event sources, exploring advanced techniques, handling errors, and implementing proper resource cleanup strategies, you can unlock the full potential of fromEvent
in RxJS. Armed with this knowledge, you will be able to build event-driven applications that respond to user interactions, create interactive interfaces, and seamlessly integrate with event-emitting systems. Harness the power of fromEvent
and unlock the full potential of event-driven reactive programming with RxJS.