Mastering the "first" Operator in RxJS: Capturing the First Emitted Value
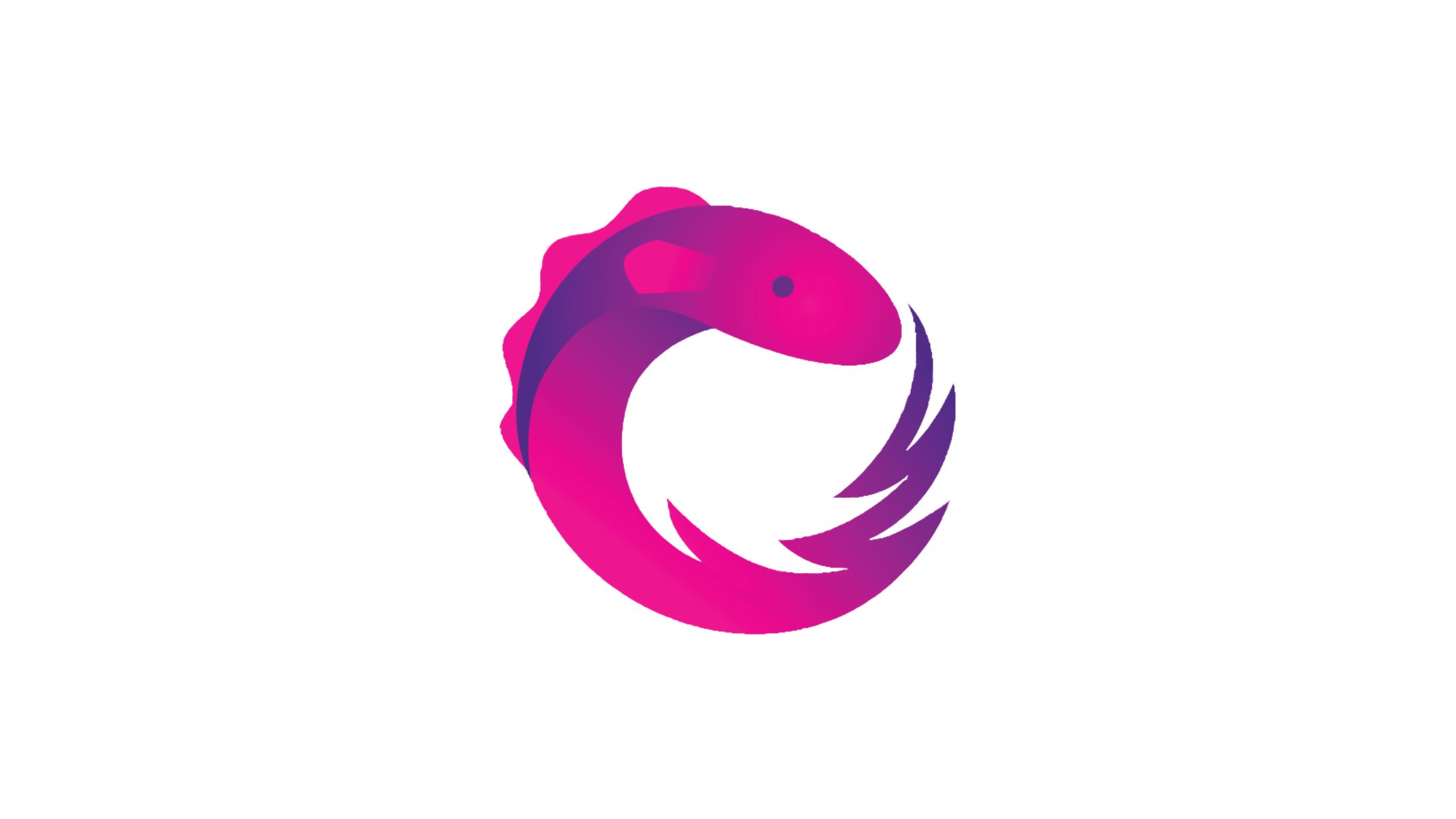
RxJS is a powerful library for reactive programming in JavaScript, and one of its fundamental operators is "first". The "first" operator allows you to capture the first emitted value from an observable. In this comprehensive guide, we will explore the "first" operator in RxJS, its syntax, and practical use cases. By mastering this operator, you will be able to accurately capture the first value emitted in a reactive data stream.
Understanding the "first" Operator
The "first" operator is used to capture the first emitted value from an observable. In this section, we will cover the basic syntax of "first" and how it works with a simple example. We will explain how the operator captures the first value and completes the emission.
import { of } from 'rxjs';
import { first } from 'rxjs/operators';
const source$ = of(1, 2, 3, 4, 5);
source$
.pipe(first())
.subscribe((value) => console.log('Captured first value:', value));
Capturing the First Value in Infinite Observables
The "first" operator can also be used with infinite observables to capture the first value before completion. We will explore examples of using "first" with observables like "interval" and "timer" to capture the first emitted value before the completion of the emission.
import { interval } from 'rxjs';
import { first } from 'rxjs/operators';
const source$ = interval(1000);
source$
.pipe(first())
.subscribe((value) => console.log('Captured first value:', value));
Combining "first" with Other Operators
The "first" operator can be combined with other operators to create more complex logic. We will cover examples of combining it with operators like "filter" and "map" to filter and transform the first captured value.
import { of } from 'rxjs';
import { first, filter, map } from 'rxjs/operators';
const source$ = of(1, 2, 3, 4, 5);
source$
.pipe(
filter((value) => value % 2 === 0),
map((value) => value * 2),
first()
)
.subscribe((value) => console.log('Captured first value:', value));
Capturing the First Value Based on Conditions
The "first" operator can be used to capture the first value based on specific conditions. We will explore examples of using "first" combined with other observables or events to capture the first value that meets a certain condition.
import { fromEvent } from 'rxjs';
import { first } from 'rxjs/operators';
const button = document.getElementById('myButton');
const click$ = fromEvent(button, 'click');
click$
.pipe(
first((event) => event.target.id === 'myButton')
)
.subscribe((event) => console.log('Captured first click:', event));
The "first" operator in RxJS allows you to capture the first emitted value from an observable. By mastering this operator, you gain control over the first value captured in a reactive data stream. In this guide, we explored the basic syntax of "first" and provided examples of its application in finite and infinite observables, combined with other operators, and based on specific conditions. Embrace the power of the "first" operator to capture the first value and enhance your skills in reactive programming with RxJS.