Mastering the "takeWhile" Operator in RxJS: Controlling Observable Emission
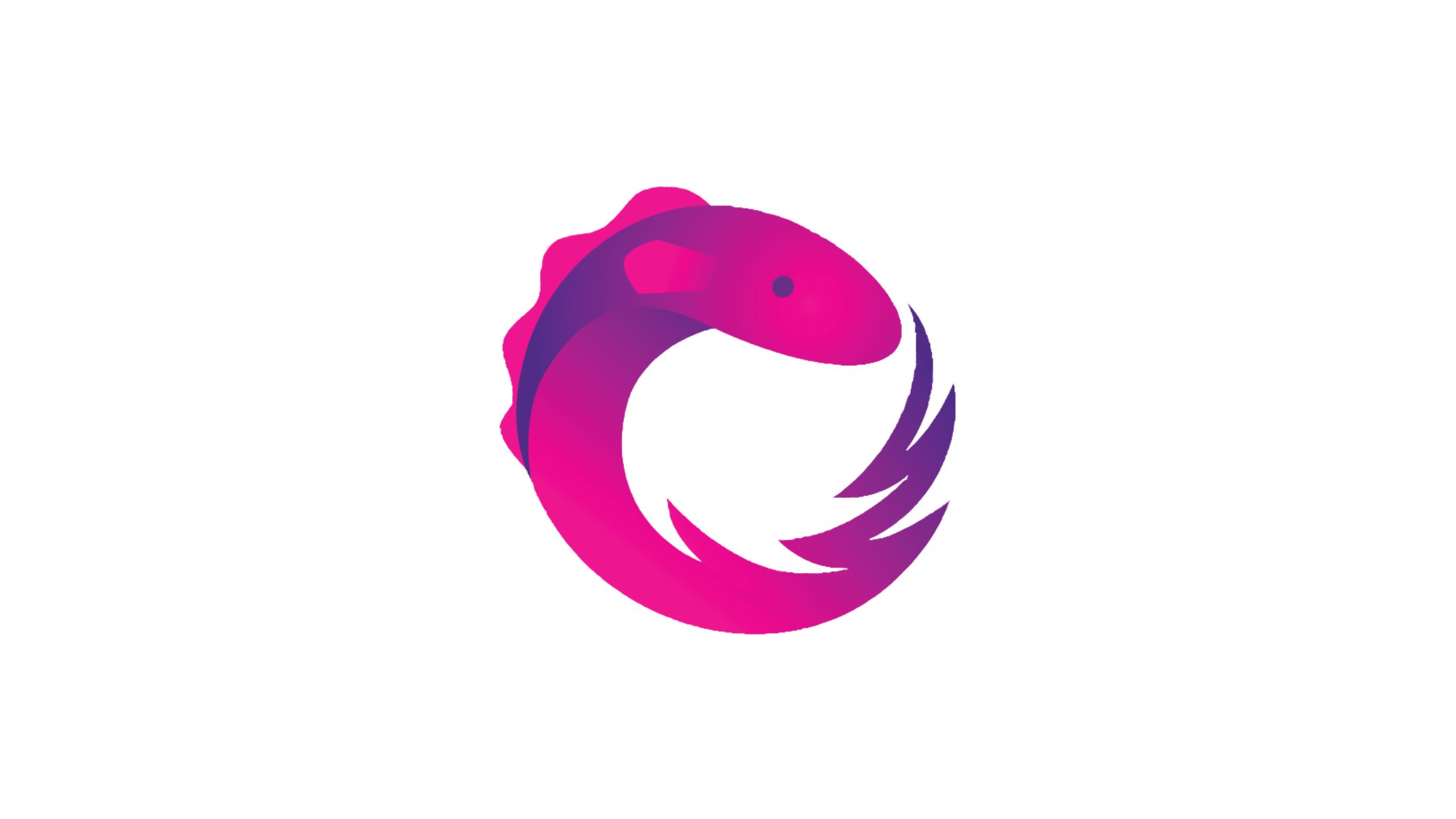
RxJS is a powerful library for reactive programming in JavaScript, and one of its fundamental operators is "takeWhile". The "takeWhile" operator allows you to take values from an observable sequence until a specified condition is no longer satisfied. In this comprehensive guide, we will explore the "takeWhile" operator in RxJS, its syntax, and practical use cases. By understanding and effectively utilizing "takeWhile", you will gain the ability to control observable emissions based on dynamic conditions in your applications.
Understanding the "takeWhile" Operator
The "takeWhile" operator is versatile and enables fine-grained control over observable emissions. We will cover the syntax of "takeWhile" and how it works by evaluating a condition for each emitted value. Furthermore, we will explain how the operator gracefully completes the subscription when the condition is no longer satisfied.
import { interval } from 'rxjs';
import { takeWhile } from 'rxjs/operators';
const source$ = interval(1000);
source$
.pipe(takeWhile((value) => value < 5))
.subscribe(
(value) => console.log('Received:', value),
(error) => console.error('Error:', error),
() => console.log('Completed')
);
Taking Values While a Condition Holds True
The primary use case of the "takeWhile" operator is to take values from an observable sequence as long as a specified condition holds true. We will explore scenarios such as extracting values from an array until a certain threshold is reached or taking values from a stream until a specific condition is no longer satisfied.
import { from, of } from 'rxjs';
import { takeWhile } from 'rxjs/operators';
const arrayData$ = from([1, 2, 3, 4, 5]);
arrayData$
.pipe(takeWhile((value) => value < 4))
.subscribe((value) => console.log('Array Item:', value));
const streamData$ = of(2, 4, 6, 8, 10);
streamData$
.pipe(takeWhile((value) => value % 2 === 0))
.subscribe((value) => console.log('Stream Value:', value));
Taking Values Until a Condition Becomes False
In addition to taking values while a condition holds true, the "takeWhile" operator can also be used to take values until a condition becomes false. We will explore examples of using "takeWhile" in combination with other operators to create complex conditions based on multiple criteria.
import { interval } from 'rxjs';
import { takeWhile, map } from 'rxjs/operators';
const source$ = interval(1000);
source$
.pipe(
takeWhile((value) => value < 10),
map((value) => value * 2)
)
.subscribe((value) => console.log('Processed Value:', value));
Dynamically Changing the Condition
One of the powerful features of the "takeWhile" operator is the ability to dynamically change the condition during runtime. We will demonstrate how to use variables, functions, or other observables to define the condition dynamically based on the emitted values.
import { interval, timer } from 'rxjs';
import { takeWhile, takeUntil } from 'rxjs/operators';
const source$ = interval(1000);
const dynamicCondition$ = timer(5000);
source$
.pipe(takeWhile(() => dynamicCondition$
))
.subscribe((value) => console.log('Dynamic Value:', value));
The "takeWhile" operator in RxJS empowers you to control observable emissions based on dynamic conditions. By leveraging its syntax, various use cases, and the ability to dynamically change conditions, you can precisely manage the values emitted by observables. With the knowledge gained in this guide, you will be well-equipped to handle scenarios where extracting values until a condition becomes false is essential. Whether it's taking values while a condition holds true, dynamically changing the condition, or combining "takeWhile" with other operators, you'll have the tools needed to handle complex data flows confidently. Embrace the power of the "takeWhile" operator and unlock precise control over observable emissions in your reactive programming with RxJS.