Mastering the "takeUntil" Operator in RxJS: Controlling Observable Emission with Another Observable
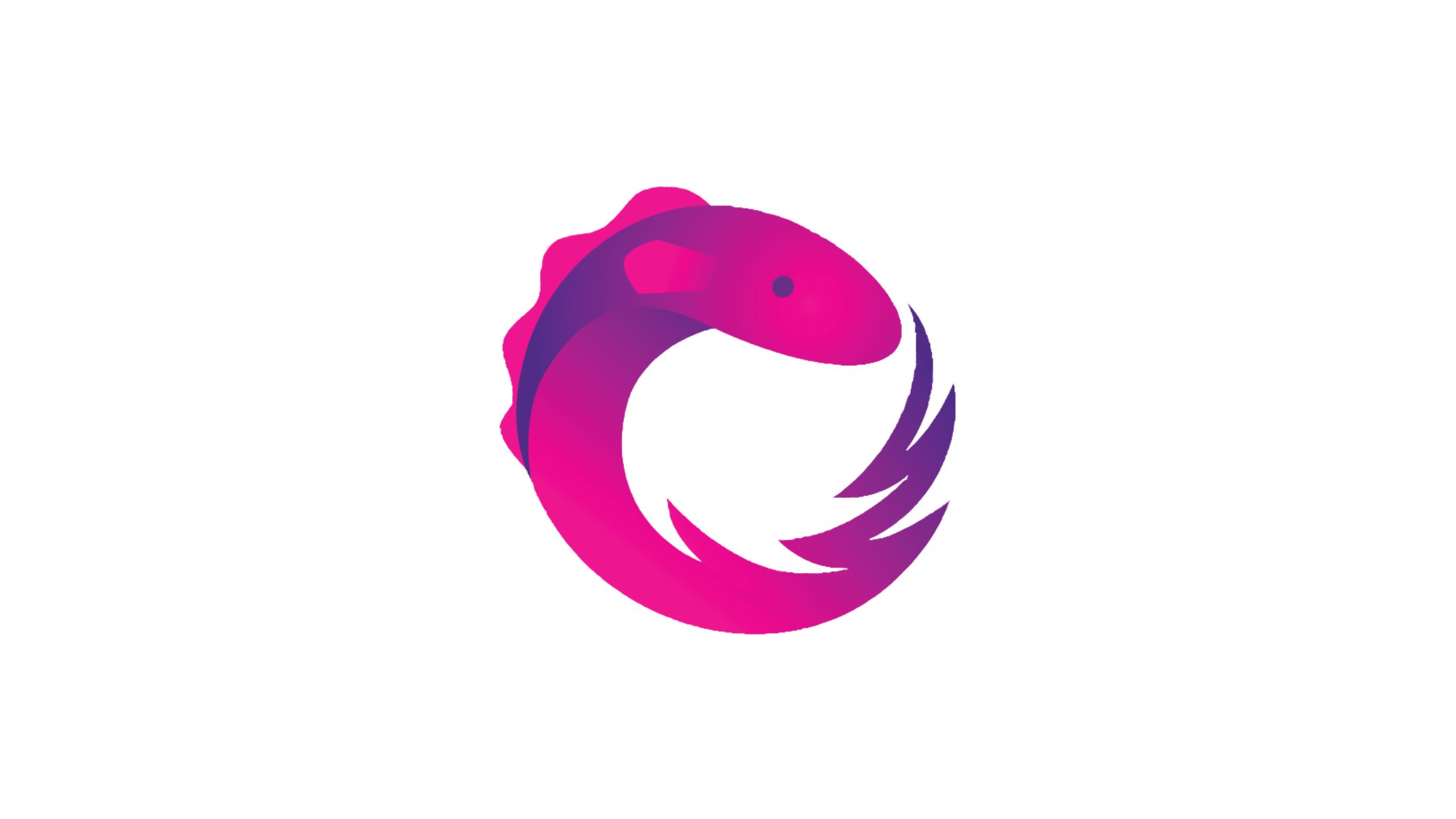
RxJS is a powerful library for reactive programming in JavaScript, and one of its essential operators is "takeUntil". The "takeUntil" operator allows you to control the emission of observables based on another observable. In this comprehensive guide, we will explore the "takeUntil" operator in RxJS, its syntax, and practical use cases. By mastering this operator, you will be able to precisely control the emission of observables by terminating it when a specific observable emits a value.
Understanding the "takeUntil" Operator
The "takeUntil" operator is a powerful tool for controlling observable emission based on another observable. In this section, we will cover the basic syntax of "takeUntil" and how it works with a simple example. We will explain how the operator stops the emission of the source observable when the provided observable emits its first value.
import { interval, timer } from 'rxjs';
import { takeUntil } from 'rxjs/operators';
const source$ = interval(1000);
const stopTimer$ = timer(5000);
source$
.pipe(takeUntil(stopTimer$))
.subscribe((value) => console.log('Emitted value:', value));
Terminating Emission Based on Dynamic Conditions
One of the powerful features of the "takeUntil" operator is the ability to terminate the emission based on dynamic conditions. We will explore use cases where you can use other observables or events to determine when the emission should be stopped.
import { interval, fromEvent } from 'rxjs';
import { takeUntil } from 'rxjs/operators';
const source$ = interval(1000);
const button = document.getElementById('stopButton');
const stopButtonClick$ = fromEvent(button, 'click');
source$
.pipe(takeUntil(stopButtonClick$))
.subscribe((value) => console.log('Emitted value:', value));
Combination with Other Operators
The "takeUntil" operator can be combined with other operators to create more complex emission control logic. We will explore examples of combining it with operators like "filter" and "debounceTime" to have even more control over when the emission should be terminated.
import { interval, fromEvent } from 'rxjs';
import { takeUntil, filter, debounceTime } from 'rxjs/operators';
const source$ = interval(1000);
const button = document.getElementById('stopButton');
const stopButtonClick$ = fromEvent(button, 'click');
source$
.pipe(
takeUntil(stopButtonClick$),
filter((value) => value % 2 === 0),
debounceTime(500)
)
.subscribe((value) => console.log('Emitted value:', value));
Terminating Emission Based on Asynchronous Operations
The "takeUntil" operator is useful for terminating the emission when an asynchronous operation completes. We will cover examples of using "takeUntil" with asynchronous operations like HTTP requests to control the emission of observables based on the completion of those operations.
import { interval } from 'rxjs';
import { takeUntil } from 'rxjs/operators';
import { HttpClient } from '@angular/common/http';
const source$ = interval(1000);
const destroy$ = new Subject();
this.http.get('https://api.example.com/data')
.pipe(takeUntil(destroy$))
.subscribe((data) => console.log('Received data:', data));
// At some point, when needed, call destroy$.next() to terminate the emission.
The "takeUntil" operator in RxJS allows you to precisely control the emission of observables based on another observable. By combining the "takeUntil" operator with other operators and manipulating observables dynamically, you can create sophisticated logic to terminate emission precisely. With the knowledge gained from this guide, you will be equipped to handle scenarios where terminating emission based on external events, dynamic conditions, or completed asynchronous operations is necessary. Embrace the power of the "takeUntil" operator and master the art of controlling observable emission in your reactive programming with RxJS.