Mastering RxJS Interval: Building Reactive Time-based Applications
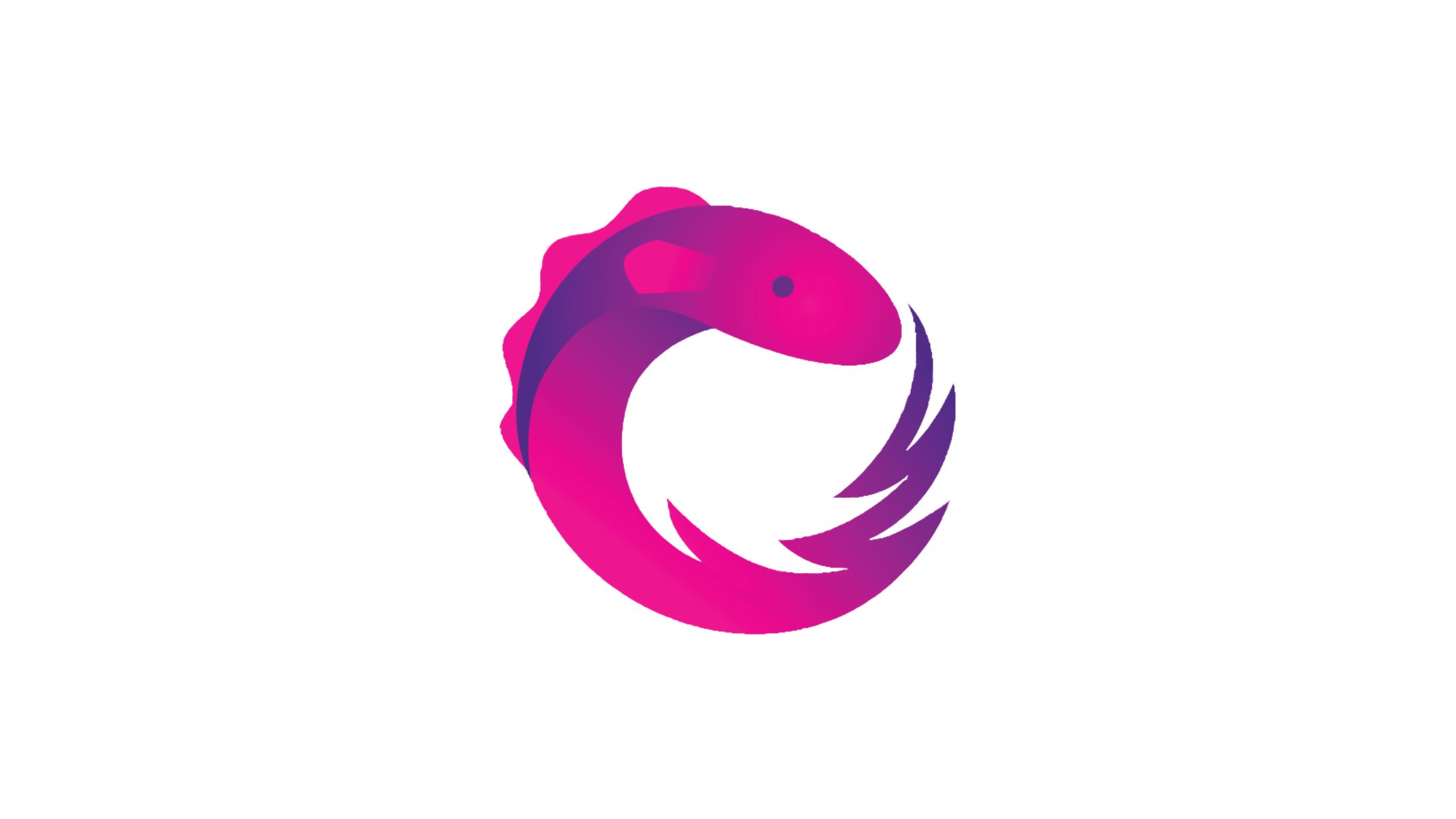
RxJS is a powerful library for reactive programming in JavaScript, and one of its key features is the interval
operator. The interval
operator allows developers to create an Observable that emits values at a regular time interval. In this guide, we will explore the interval operator in RxJS, its syntax, and various use cases. We will dive deep into understanding how to leverage interval for building time-based applications and explore advanced techniques and best practices along the way.
Understanding RxJS Interval
The interval
operator is part of the core set of operators in RxJS. It creates an Observable that emits incremental numbers at a specified time interval. The syntax for interval is straightforward, using a single argument to define the time interval
in milliseconds. For example, interval(1000)
emits a value every second.
import { interval } from 'rxjs';
const subscription = interval(1000).subscribe((value) => {
console.log(value);
});
Creating and Subscribing to Interval Observables
To use interval
, we need to create an Observable and subscribe to it. We'll explore how to create an interval
Observable using the interval
operator and then subscribe to it to start receiving emitted values. We'll cover subscribing with a callback, handling emissions, and managing subscriptions effectively.
import { interval } from 'rxjs';
const subscription = interval(1000).subscribe({
next: (value) => {
console.log(value);
},
complete: () => {
console.log('Complete!');
},
error: (err) => {
console.error('Error:', err);
},
});
// Unsubscribing after 10 sec
setTimeout(() => subscription.unsubscribe(), 10000);
Transforming Interval Emissions
While interval
emits simple incremental numbers, we can leverage other RxJS operators to transform these emissions into more meaningful values. We'll explore operators like map, filter, and take to manipulate the interval emissions and customize the emitted values based on our requirements.
import { interval } from 'rxjs';
import { map, filter, take } from 'rxjs/operators';
const subscription = interval(1000)
.pipe(
map((value) => value * 2),
filter((value) => value % 3 === 0),
take(5)
)
.subscribe((value) => {
console.log(value);
});
Scheduling and Controlling Intervals
RxJS provides various scheduling options to control how intervals are emitted. We'll explore the scheduler argument of the interval
operator, allowing us to control the timing and execution context of the interval
emissions. We'll also cover techniques like pausing, resuming, and restarting intervals using operators like pausable
, takeUntil
, and repeat
.
import { interval, asyncScheduler } from 'rxjs';
import { takeUntil, repeat } from 'rxjs/operators';
const stopSignal$ = new Subject();
interval(1000, asyncScheduler)
.pipe(takeUntil(stopSignal$), repeat())
.subscribe((value) => {
console.log(value);
});
// Pause and Resume example
setTimeout(() => {
stopSignal$.next({});
}, 5000);
setTimeout(() => {
stopSignal$.complete();
}, 7000);
Advanced Techniques and Use Cases
In this section, we'll delve into advanced techniques and real-world use cases of interval
in RxJS. We'll explore scenarios like polling data from APIs, creating animations, scheduling tasks, and building reactive timers and counters. We'll also discuss how to handle errors and gracefully terminate interval
observables.
RxJS interval
is a versatile operator that enables developers to build reactive time-based applications in JavaScript. By understanding its syntax, transforming interval emissions, controlling intervals, and exploring advanced techniques, you can harness the full power of interval in RxJS. Armed with this knowledge, you can confidently incorporate time-based functionality into your applications, providing users with responsive and dynamic experiences. Experiment with interval
and explore its possibilities to unlock new dimensions of reactive programming with RxJS.
Check and customize the examples here