Mastering RxJS scan: Managing State Transitions with Confidence
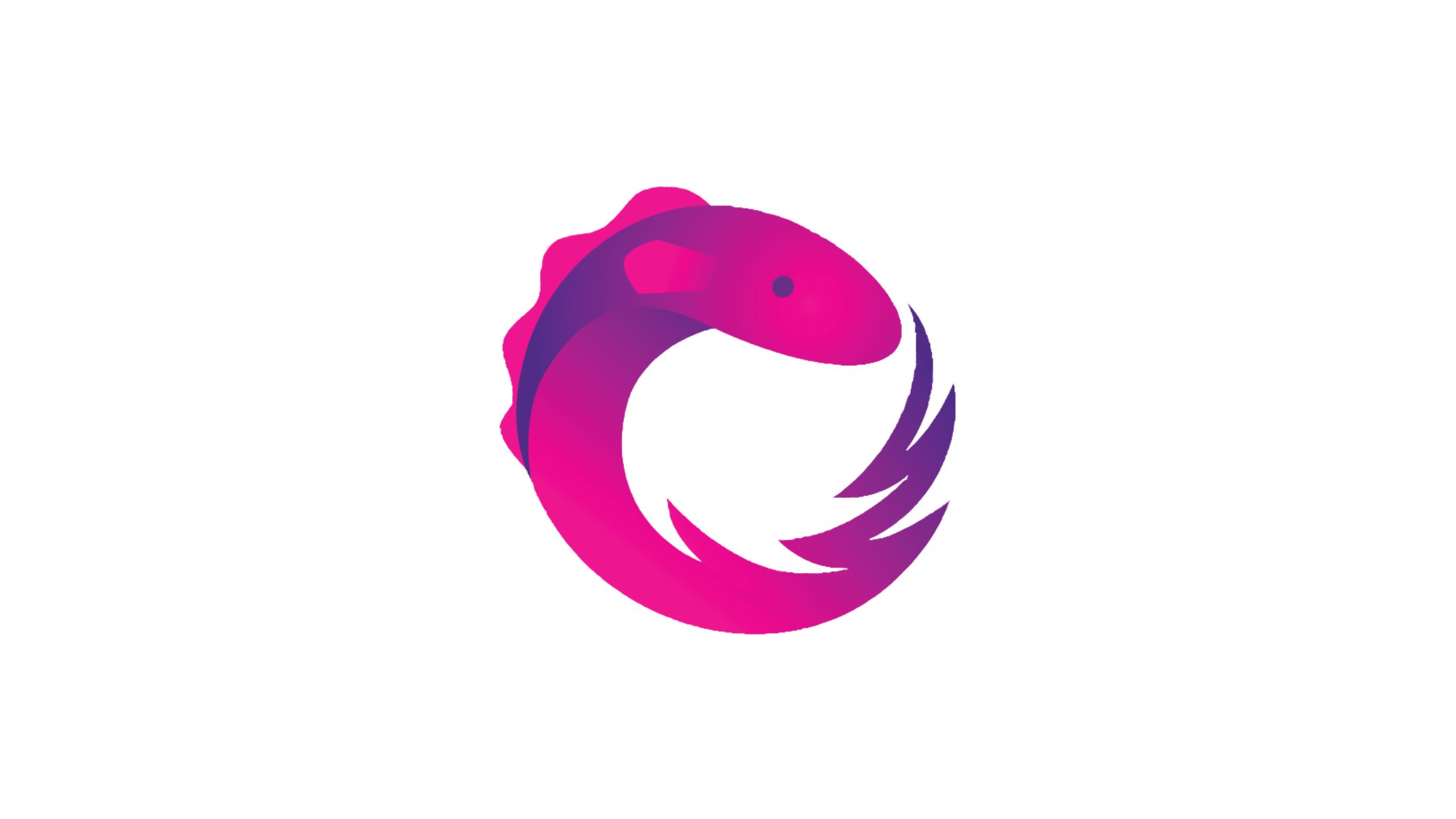
RxJS is a powerful library for reactive programming in JavaScript, and one of its essential operators is scan
. The scan
operator allows developers to manage state transitions in a predictable and controlled manner. In this guide, we will explore the scan
operator in RxJS, its syntax, and various use cases. By understanding how to effectively use scan
, you will gain the ability to handle complex state changes and build more robust applications.
Understanding RxJS scan
The scan
operator in RxJS is designed to accumulate values over time and emit intermediate results. We will cover the syntax of scan
and its parameters, such as the accumulator function. Additionally, we will discuss the concept of state management and how scan
helps in tracking and transforming state values.
import { from } from 'rxjs';
import { scan } from 'rxjs/operators';
const numbers$ = from([1, 2, 3, 4, 5]);
numbers$
.pipe(scan((accumulator, currentValue) => accumulator + currentValue, 0))
.subscribe((result) => {
console.log('Accumulated sum:', result);
});
Managing State with Scan
State management is a crucial aspect of many applications, and scan
provides a powerful tool for handling state transitions. We will explore how scan
can be used to manage state in different scenarios, such as managing a shopping cart, tracking user authentication status, and implementing undo/redo functionality. By leveraging scan
, you can easily maintain and update complex application states.
import { fromEvent } from 'rxjs';
import { scan } from 'rxjs/operators';
const addButton = document.querySelector('#addButton');
const removeButton = document.querySelector('#removeButton');
const addClick$ = fromEvent(addButton, 'click');
const removeClick$ = fromEvent(removeButton, 'click');
addClick$
.pipe(scan((count) => count + 1, 0))
.subscribe((count) => {
console.log('Items in cart:', count);
});
removeClick$
.pipe(scan((count) => Math.max(count - 1, 0), 0))
.subscribe((count) => {
console.log('Items in cart:', count);
});
Complex State Transitions
In some cases, state transitions can involve more complex operations, such as merging multiple sources of data or applying conditional logic. We will explore advanced techniques with scan
to handle such scenarios, including combining multiple streams with zip
and implementing conditional state updates with switchMap
. These techniques will empower you to handle intricate state transitions with ease.
import { fromEvent } from 'rxjs';
import { scan, zip, switchMap } from 'rxjs/operators';
const widthInput = document.querySelector('#widthInput');
const heightInput = document.querySelector('#heightInput');
const calculateButton = document.querySelector('#calculateButton');
const width$ = fromEvent(widthInput, 'input').pipe(scan((_, event) => event.target.value, ''));
const height$ = fromEvent(heightInput, 'input').pipe(scan((_, event) => event.target.value, ''));
calculateButton
.pipe(
switchMap(() => zip(width$, height$)),
scan((accumulator, [width, height]) => {
const area = width * height;
return [...accumulator, area];
}, [])
)
.subscribe((areas) => {
console.log('Calculated areas:', areas);
});
Optimizing Performance with Scan
The scan
operator can sometimes accumulate a large amount of data over time, leading to potential performance issues. We will discuss strategies to optimize performance when using scan
, such as using the distinctUntilChanged
operator to avoid emitting duplicate state values and leveraging the shareReplay
operator to cache and share intermediate results. These techniques will help you build efficient and responsive applications.
Conclusion
The scan
operator in RxJS is a versatile tool for managing state transitions in a reactive manner. By understanding its syntax, applying it to different scenarios, managing complex state changes, and optimizing performance, you can harness the full power of scan
in RxJS. With this knowledge, you will be equipped to handle state management challenges, build more robust applications, and create seamless user experiences. Embrace the capabilities of scan
and unlock the potential of managing state transitions with confidence in reactive programming with RxJS.