Mastering RxJS throttleTime: Controlling Event Emission with Precision
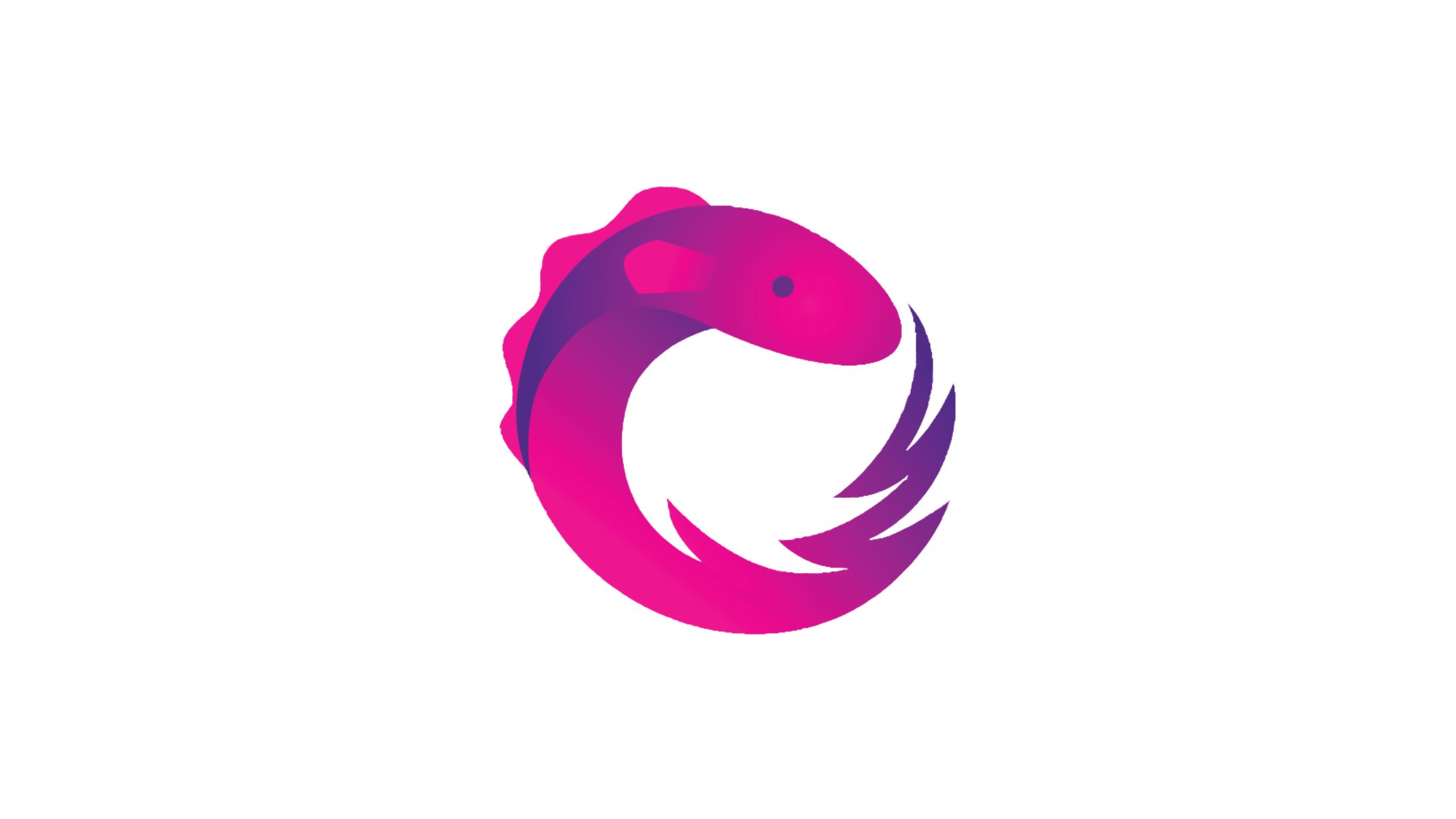
RxJS is a powerful library for reactive programming in JavaScript, and one of its essential operators is throttleTime
. The throttleTime
operator allows developers to control the rate at which events are emitted by an Observable. In this guide, we will explore the throttleTime
operator in RxJS, its syntax, and various use cases. By understanding how to effectively use throttleTime
, you will be able to optimize event processing and create smoother user experiences.
Understanding RxJS throttleTime
The throttleTime
operator is designed to control the rate at which events are emitted by an Observable. We will cover the syntax of throttleTime
and its parameters, such as the time duration for throttling events. Additionally, we will discuss the concept of throttling and how it helps prevent an excessive number of events from overwhelming a system.
import { fromEvent } from 'rxjs';
import { throttleTime } from 'rxjs/operators';
const button = document.querySelector('#myButton');
const buttonClick$ = fromEvent(button, 'click');
buttonClick$
.pipe(throttleTime(1000))
.subscribe(() => {
console.log('Button clicked!');
});
Throttling DOM Events
DOM events often occur at high frequencies, and throttling can help reduce unnecessary event processing. We will demonstrate how to apply throttleTime
to different DOM events such as clicks, mouse movements, and key presses. This will ensure that the events are emitted at a controlled rate, improving performance and responsiveness.
import { fromEvent } from 'rxjs';
import { throttleTime } from 'rxjs/operators';
const button = document.querySelector('#myButton');
const input = document.querySelector('#myInput');
const buttonClick$ = fromEvent(button, 'click');
const inputKeyup$ = fromEvent(input, 'keyup');
buttonClick$
.pipe(throttleTime(1000))
.subscribe(() => {
console.log('Button clicked!');
});
inputKeyup$
.pipe(throttleTime(500))
.subscribe((event) => {
console.log('Key pressed:', event.key);
});
Throttling HTTP Requests
When making HTTP requests, it's often necessary to limit the frequency to prevent overwhelming the server or wasting resources. We will demonstrate how throttleTime
can be applied to throttle HTTP requests by using RxJS's ajax
function. This technique ensures that requests are made at a controlled rate, reducing unnecessary network traffic and optimizing resource usage.
import { fromEvent } from 'rxjs';
import { throttleTime } from 'rxjs/operators';
import { ajax } from 'rxjs/ajax';
const button = document.querySelector('#myButton');
const buttonClick$ = fromEvent(button, 'click');
buttonClick$
.pipe(throttleTime(1000))
.subscribe(() => {
ajax.getJSON('https://api.example.com/data')
.subscribe((response) => {
console.log('Data received:', response);
});
});
Customizing Throttling Behavior
The throttleTime
operator provides flexibility for customizing the throttling behavior based on specific requirements. We will explore additional parameters such as leading
and trailing
to control the emission of the first and last event within a throttle duration. We will also discuss the use of the scheduler
parameter to fine-tune the timing of event emissions.
import { fromEvent, asyncScheduler } from
'rxjs';
import { throttleTime } from 'rxjs/operators';
const button = document.querySelector('#myButton');
const buttonClick$ = fromEvent(button, 'click');
buttonClick$
.pipe(throttleTime(1000, asyncScheduler, { leading: true, trailing: true }))
.subscribe(() => {
console.log('Button clicked!');
});
Real-World Use Cases
In this section, we will explore real-world use cases where throttleTime
is invaluable. We will cover scenarios such as rate limiting user interactions, handling scroll events, optimizing search suggestions, and preventing multiple form submissions. By examining these practical examples, you will gain a deeper understanding of how to apply throttleTime
to create more efficient and responsive applications.
The RxJS throttleTime
operator is a powerful tool for controlling the emission rate of events in a reactive manner. By understanding its syntax, applying it to various types of events, customizing the throttling behavior, and exploring real-world use cases, you can harness the full potential of throttleTime
in RxJS. With this knowledge, you'll be able to optimize event processing, prevent excessive event emissions, and create smoother user experiences in your applications. Embrace the precision of throttleTime
and unlock the power of event throttling in reactive programming with RxJS.