Mastering the Take Operator in RxJS: Managing Observables with Precision
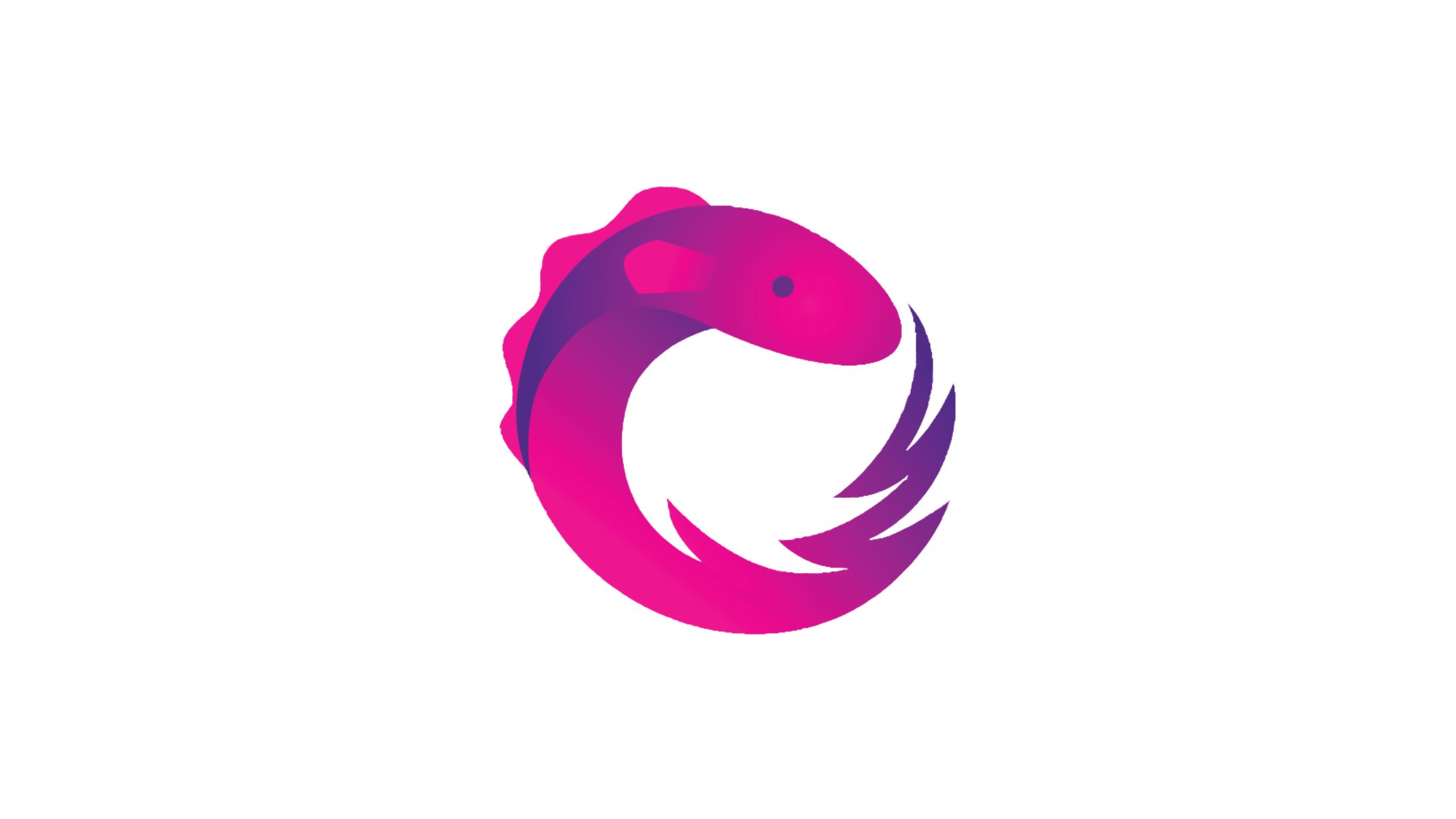
RxJS is a powerful library for reactive programming in JavaScript, and one of its fundamental operators is take
. The take
operator allows you to extract a specific number of values from an observable sequence and then automatically unsubscribe. In this comprehensive guide, we will explore the take
operator in RxJS, its syntax, and various practical use cases. By understanding and effectively utilizing take
, you will gain the ability to manage observables precisely and control the flow of data in your applications.
Understanding the Take Operator
The take
operator is straightforward but essential. We will cover the syntax of take
and its parameter, the number of values to be extracted. Additionally, we will explain how the take
operator ensures that you only receive the desired number of values and then automatically completes the subscription.
import { interval } from 'rxjs';
import { take } from 'rxjs/operators';
const source$ = interval(1000);
source$
.pipe(take(5))
.subscribe(
(value) => console.log('Received:', value),
(error) => console.error('Error:', error),
() => console.log('Completed')
);
Taking a Slice of Data
The take
operator is particularly useful when you want to extract a specific slice of data from a potentially infinite stream. We will explore scenarios like extracting the first n
items from an array or limiting the number of HTTP responses received from a server.
import { from, of } from 'rxjs';
import { take } from 'rxjs/operators';
const arrayData$ = from([1, 2, 3, 4, 5]);
arrayData$
.pipe(take(3))
.subscribe((value) => console.log('Array Item:', value));
const serverResponse$ = of('Response 1', 'Response 2', 'Response 3', 'Response 4', 'Response 5');
serverResponse$
.pipe(take(2))
.subscribe((response) => console.log('Server Response:', response));
Taking Until a Condition is Met
The take
operator can be combined with other operators to extract values until a certain condition is met. We will explore how to use takeWhile
and takeUntil
to take values based on specific criteria or until another observable emits a value.
import { interval, timer } from 'rxjs';
import { takeWhile, takeUntil } from 'rxjs/operators';
const source1$ = interval(1000);
source1$
.pipe(takeWhile((value) => value < 5))
.subscribe((value) => console.log('Value from takeWhile:', value));
const source2$ = interval(1000);
const timer$ = timer(5000);
source2$
.pipe(takeUntil(timer$))
.subscribe((value) => console.log('Value from takeUntil:', value));
Taking Data Periodically
The take
operator is handy for extracting data periodically from an observable sequence. We will demonstrate how to use take
in combination with the interval
and timer
observables to capture values at regular intervals or for a specific duration.
import { interval, timer } from 'rxjs';
import { take } from 'rxjs/operators';
const source3$ = interval(1000);
source3$
.pipe(take(3))
.subscribe((value) => console.log('Interval Value:',
value));
const timerSource$ = timer(2000, 1000);
timerSource$
.pipe(take(5))
.subscribe((value) => console.log('Timer Value:', value));
Combining Take with Other Operators
The take
operator can be combined with various other operators to achieve complex data extraction scenarios. We will explore examples of using take
in combination with filter
, map
, and concat
operators to extract specific values from an observable sequence.
import { interval } from 'rxjs';
import { take, filter, map, concatMap } from 'rxjs/operators';
const source$ = interval(1000);
source$
.pipe(
take(5),
filter((value) => value % 2 === 0),
map((value) => value * 10),
concatMap((value) => of(value).pipe(delay(500)))
)
.subscribe((value) => console.log('Processed Value:', value));
Conclusion
The take
operator in RxJS empowers you to precisely manage observables and control the flow of data. By understanding its syntax, practical applications, and how to combine it with other operators, you can extract the desired number of values from observable sequences efficiently. With the knowledge gained from this guide, you'll be equipped to handle various scenarios where precise data extraction is crucial. Whether it's extracting a slice of data, taking values until a condition is met, capturing data periodically, or combining take
with other operators, you'll have the tools to handle complex data flows with confidence. Embrace the power of the take
operator and unlock precise observables management in your reactive programming with RxJS.